jQuery endless looped slider
Here’s another variation on a jQuery slider, this time with the images in an endless loop. Tested in IE6/7/8, Firefox 3, Chrome, Opera 9/10, Safari 4, all on Windows XP.
The demo


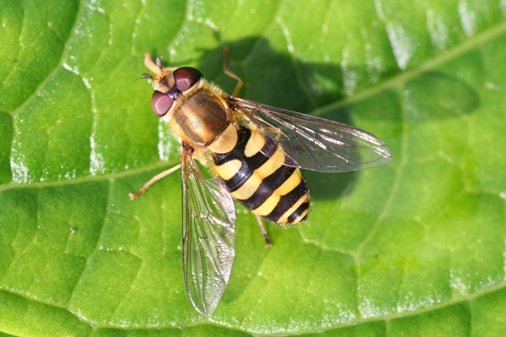
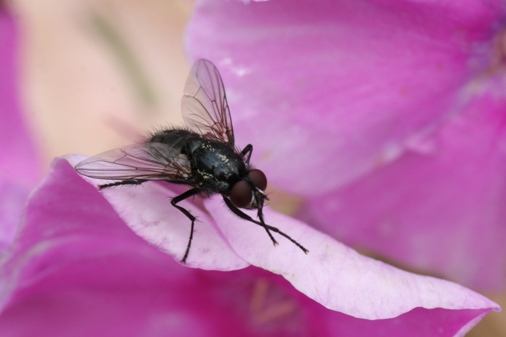
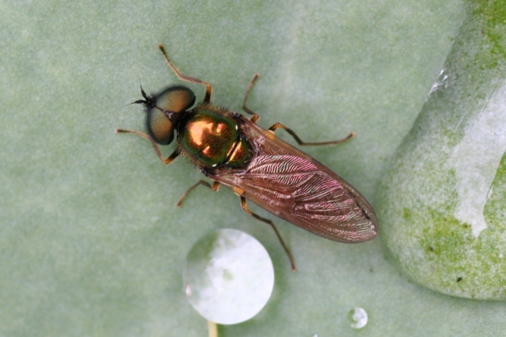
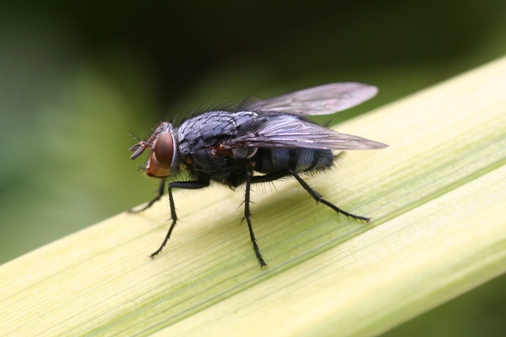
The code
Here’s my example html:
<div id="slider_box"> <div id="endless_slider_arrows"> <img id="endless_slider_left" src="/images/misc/left_arrow.gif" alt="Scroll left" title="Scroll left" width="56" height="56"/> <img id="endless_slider_right" src="/images/misc/right_arrow.gif" alt="Scroll right" title="Scroll right" width="56" height="56"/> </div> <div id="slider_images"> <img class="active" src="/path/to/image1.jpg"/> <img src="/path/to/image2.jpg"/> </div> </div>
Here’s the necessary css – just to show the important bits – you’ll need to add some more to position and style as required:
#slider_box{border:1px solid #303030;position:relative;height:337px;width:506px;overflow:hidden} #slider_images{position:absolute;left:0;top:0} #slider_box img{float:left}
And here’s the javascript:
$(document).ready(function(){ var $imgWidth = $('#slider_images img').first().outerWidth();//read the image width var $imgCount = $('#slider_images img').length;//count the images $('#slider_images').width($imgWidth*($imgCount+2));//set the width of the container to the number of images - plus 2 to account for the cloned images $('#slider_images img').first().addClass('endless_slider_first');//identify the first and last images $('#slider_images img').last().addClass('endless_slider_last'); $('.endless_slider_first').clone().appendTo('#slider_images');//clone the first image and put it at the end $('.endless_slider_last').clone().prependTo('#slider_images');//clone the last image and put it at the front $('#slider_images').css({'left':-1*$imgWidth+'px'});//reset the slider so the first image is still visible $('#endless_slider_right').click(function(){ $('#slider_images').stop(true,true); //complete any animation still running - in case anyone's a bit click happy... var $newLeft = $('#slider_images').position().left-(1*$imgWidth);//calculate the new position which is the current position minus the width of one image $('#slider_images').animate({'left':$newLeft+'px'},function(){//slide to the new position... if (Math.abs($newLeft) == (($imgCount+1)*$imgWidth)) //...and if the slider is displaying the last image, which is the clone of the first image... { $('#slider_images').css({'left':-1*$imgWidth+'px'});//...reset the slider back to the first image without animating } }); return false; }); $('#endless_slider_left').click(function(){ $('#slider_images').stop(true,true); //complete any animation still running var $newLeft = $('#slider_images').position().left+(1*$imgWidth);//calculate the new position which is the current position plus the width of one image $('#slider_images').animate({'left':$newLeft+'px'},function(){//slide to the new position if (Math.abs($newLeft) == (0)) //if the slider is displaying the first image, which is the clone of the last image { $('#slider_images').css({'left':-($imgCount)*$imgWidth+'px'});//reset the slider back to the last image without animating } }); return false; }); })
The logic
The slider container, here #slider_box
has overflow hidden. The images are contained within an absolutely positioned div, #slider_images
which has its width set to the number of images, plus two, times the image width. I’m then cloning the first image, and placing it at the end of the images, and cloning the last image and placing it before the first image.
I’m animating the left and right click events by changing the left position of #slider_images
by the width of an image. Then, at the completion of each animation, I’m checking the left position to see if I’m at the end of the images, displaying a cloned image, and if so swapping back to the original image. So, if I’m displaying the last image, which is the cloned first image, I change the position again, without animating, back to displaying the orginal non-cloned first image. This swap is invisible to the user and hence we give the appearance of an infinite loop.
Can I use this on my site?
Probably. See my policies on using code from this site.
Have a look at this page, for looping a background image. The javascript is virtually identical, you just need to amend your css.
I want to make a full page(windows full width & full height) slider with looping images. Please help
Have a look at this example for a similar responsive slideshow.
thank you very much and how can i responsive this slideshow i need to heve this slide show in responsive mode thank you again
You can see the html if you view the source of the page.
Very useful. But I coul’d get it. html is also missing. How can ı get all?
Thank you very much this was exactly looking for !
Absolute super. Exactly what I needed. THX a lot
Awesome tutorial, javascript comments are super helpful! Thanks!
Thank you very much! Excellent explanation, that help me a lot!
thank you very much! This help me alot.
I’m going mark this page with Google plusone button.
Thank You,
this is exactly what I was looking for. Nicely explained, just read carefully comments. The trick was to clone first and last image and jump to original image by setting position without animating.